In this tutorial, I will show you how to create multi select dropdown in angular project. I going to load the dropdown data from the api. For this I have created a service which has a getDropDownText method which I can fetch the selected value text.
Component:
Creating Service in Angular:
import * as _ from 'lodash';
public URL: any="https://jsonplaceholder.typicode.com/users";
constructor(private http:HttpClient) { }
getUsers() {
return this.http.get(this.URL)
}
getDropDownText(id, object){
const selObj = _.filter(object, function (o) {
return (_.includes(id,o.id));
});
return selObj;
}
Component:
public users:any;
constructor( private _service:StudentService ) { }
get(){
this._service.getUsers().subscribe(res=>
this.users=res);
}
mySelect = [];
selectedValue: any;
selectChange() {
this.selectedValue = this._service.getDropDownText(this.mySelect, this.users);
}
Html Code:
<h2>Multiple Dropdown Selections</h2>
<select name="my-select" [(ngModel)]="mySelect" (change)="selectChange()" multiple="multiple"> <option [value]="item.id" *ngFor="let item of users"> {{item.name}} </option></select>
<h4>Selected Values</h4>
<ng-container> <div> Selected: <ul> <li *ngFor="let SelVal of selectedValue">{{SelVal.name}}</li> </ul> </div></ng-container><ng-template #elseTemplate> <div> Selected: {{selectedValue}} </div></ng-template>
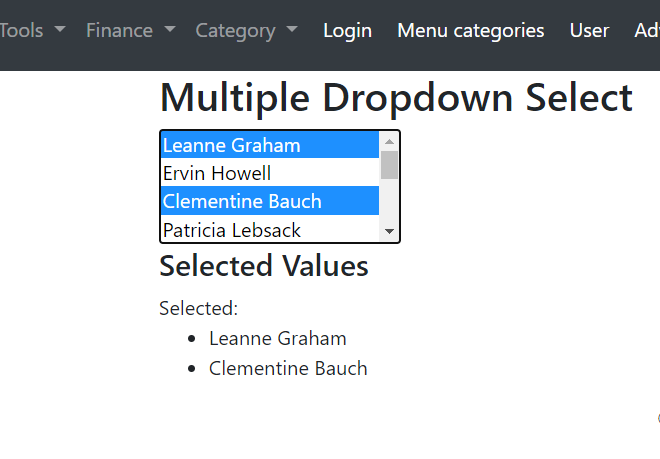
Video Guide:
<h2>Multiple Dropdown Selections</h2>
<select name="my-select" [(ngModel)]="mySelect" (change)="selectChange()" multiple="multiple">
<option [value]="item.id" *ngFor="let item of users">
{{item.name}}
</option>
</select>
<h4>Selected Values</h4>
<ng-container>
<div>
Selected:
<ul>
<li *ngFor="let SelVal of selectedValue">{{SelVal.name}}</li>
</ul>
</div>
</ng-container>
<ng-template #elseTemplate>
<div>
Selected: {{selectedValue}}
</div>
</ng-template>
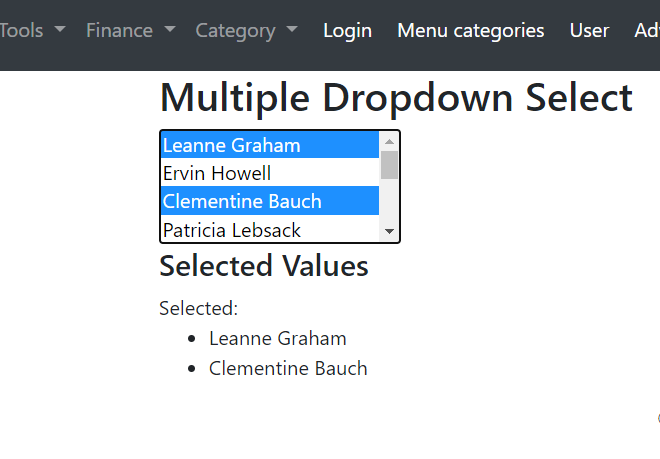
Post your comments / questions
Recent Article
- How to create custom 404 error page in Django?
- Requested setting INSTALLED_APPS, but settings are not configured. You must either define..
- ValueError:All arrays must be of the same length - Python
- Check hostname requires server hostname - SOLVED
- How to restrict access to the page Access only for logged user in Django
- Migration admin.0001_initial is applied before its dependency admin.0001_initial on database default
- Add or change a related_name argument to the definition for 'auth.User.groups' or 'DriverUser.groups'. -Django ERROR
- Addition of two numbers in django python
Related Article