In this tutorial I will show you addition of two numbers using java in android studio.
Java Program to Add two Numbers:
Open Android Studio and create a new project.
DESIGN CODE:
In the activity_main.xml, add the following code:
Activity_main.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:gravity="center_horizontal" android:layout_marginTop="50dp" android:textSize="20dp" android:text="Enter the First Number:" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:gravity="center_horizontal" android:layout_marginTop="25dp" android:ems="10" android:id="@+id/txtFirstNo" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:gravity="center_horizontal" android:layout_marginTop="50dp" android:textSize="20dp" android:text="Enter the Second Number:" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:gravity="center_horizontal" android:layout_marginTop="25dp" android:ems="10" android:id="@+id/txtSecondNo" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/btnAdd" android:text="Add" android:layout_gravity="center_horizontal" android:gravity="center_horizontal" android:layout_marginTop="25dp" android:onClick="Calculate" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtResult" android:layout_gravity="center_horizontal" android:gravity="center_horizontal" android:layout_marginTop="50dp" android:textSize="20dp" /> </LinearLayout>
MainActivity.java:
In the MainActivity.java file, add the following code:
package com.infinetsoft.addition; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private EditText txtFirstNo; private EditText txtSecondNo; private TextView txtResult; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); txtFirstNo=(EditText) findViewById(R.id.txtFirstNo); txtSecondNo=(EditText) findViewById(R.id.txtSecondNo); txtResult=(TextView) findViewById(R.id.txtResult); } public void Calculate(View v){ Float first= Float.parseFloat( txtFirstNo.getText().toString()) ; Float second= Float.parseFloat( txtSecondNo.getText().toString()) ; Float result=first+second; txtResult.setText(result.toString()); } }
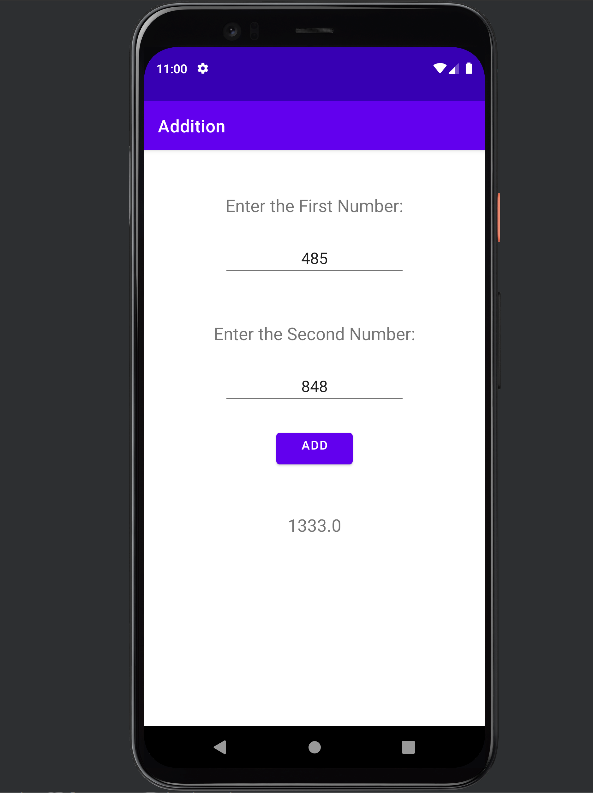
Post your comments / questions
Recent Article
- How to create custom 404 error page in Django?
- Requested setting INSTALLED_APPS, but settings are not configured. You must either define..
- ValueError:All arrays must be of the same length - Python
- Check hostname requires server hostname - SOLVED
- How to restrict access to the page Access only for logged user in Django
- Migration admin.0001_initial is applied before its dependency admin.0001_initial on database default
- Add or change a related_name argument to the definition for 'auth.User.groups' or 'DriverUser.groups'. -Django ERROR
- Addition of two numbers in django python
Related Article