In this tutorial I will show you how to convert rgb color tuple to Hexa decimal string using Python.
PYTHON CODE:
import numpy as np import matplotlib.pyplot as plt from sklearn.cluster import KMeans from PIL import Image image = Image.open('mobile.jpg') image_np = np.array(image) w, h, d = image_np.shape pixels = image_np.reshape(-1, 3) n_colors = 10 kmeans = KMeans(n_clusters=n_colors, random_state=42, n_init=10) kmeans.fit(pixels) colors_rgb = kmeans.cluster_centers_.astype(np.uint8) #Convert rgb value to hex string hex_colors= ['#{:02x}{:02x}{:02x}'.format(int(r),int(g),int(b)) for r,g,b in colors_rgb] print("Hex_decimal color values:") for hex_color in hex_colors: print(hex_color)OUTPUT:
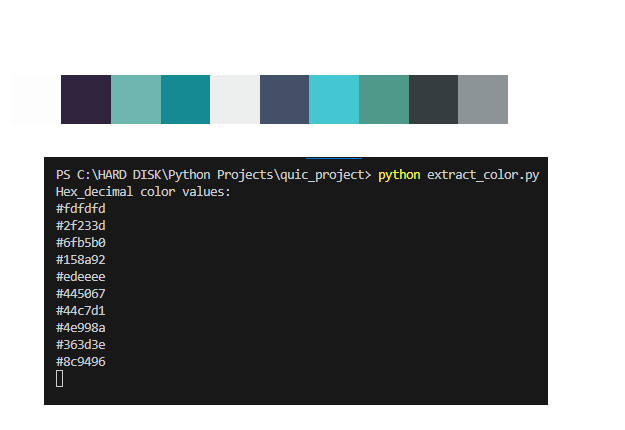
VIDEO GUIDE:
Post your comments / questions
Recent Article
- FieldError: Cannot resolve keyword 'id' into field in Django project
- How to hide the ID field from the Django admin?
- It is impossible to add a non nullable field without specifying a default. Django error
- ImportError: cannot import name 'url' from 'django.conf.urls' - Django Error
- How to Enable Virtualization in BIOS Security Settings in Intel Processors For Android Studio?
- Dependency 'androidx.activity:activity:1.8.0' requires libraries and applications that depend on it.
- AttributeError: 'NoneType' object has no attribute 'get_text' - Python
- ModuleNotFoundError: No module named 'openpyxl' - Python
Related Article